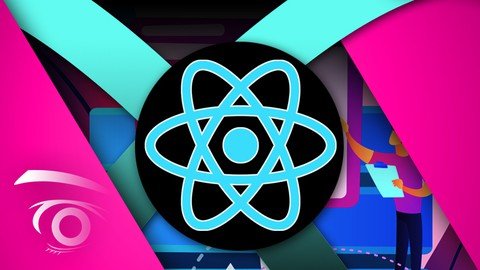
Published 8/2022
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz
Language: English | Size: 4.82 GB | Duration: 10h 24m
Learn React JS through a Series of Hands-On Projects. Build an E-Commerce Site, Calculator, and Connect4 Game.
What you'll learn
What is React?
The Tools Needed to Build React Projects
Introduction to Code Pen
Functional Components in React
Building a Fully Functional Calculator in React
Using React Props
Debugging in React
Using the React State Hook
Introduction to JSX
Styling React Projects
React Events
Building a Single and Multi-Player Connect-4 Clone Game with AI
Introduction to JSON Server
Using Fetch API
Building a Custom E-Commerce Site in React
Building an Integrated Search Function using React
Validating Forms in React
Requirements
Basic Computer Skills
No programming experience needed. You will learn everything you need to know.
Description
Welcome to the Complete React Certification course. This course offers a comprehensive guide into one of the most powerful, modern jаvascript libraries available - ReactJS. Whether you are completely new to React, or you've dabbled with it in the past, one thing's for sure - You've likely interacted with a number of web applications that use the React library for building out user interface components. Some of the most well known examples include Netflix, Facebook, and Airbnb. Developed and maintained by Meta along with a community of independent developers, React remains free and open-source. React is a component based library built entirely on jаvascript, which makes it perfect for designing complex UI's. With React, developers can build encapsulated components that efficiently manage their own state and render UI updates specifically when data changes. For example, think of the automated content refresh feature you see on a twitter feed, or Facebook like button. Here, the state of the UI component changes on the page without having to manually refresh when the data is updated. This is just one small, yet powerful UI feature built into React.This course starts with exploring the foundations of React and its core use cases. We offer a concise definition of what React is and what it's not. From there, we explore the tools needed to get started along with an introduction into JSX, and Functional React components. Through a complete hands-on project you will learn a number of important concepts including react props, callbacks, onclick Events and passing parameters within callback functions. By the end of module one you will have built a fully functional calculator, complete with display and numerical operators. We will also cover important topics such as the React State Hook, and Debugging.In the second module students build out a multiplayer Connect-4 clone, with AI integration. Here we start with styling the individual game board components followed by advanced onclickEvents. This includes global, dynamic and inline styling. From there, we move on to passing props, destructing, and React children. Students will explore the React key property, along with Lifecycle events, game initialization, CSS variables, and conditional rendering. By the end, you will have built a complete Connect-4 game capable of automatically determining the winner in both a multi-player and AI based single player setting.In module three we further unleash the power of React by building out a complete e-commerce site with multiple product categories, a product showcase, shopping cart feature, and much more. Here, we introduce a number of integral new concepts including: JSON server, Fetch API, and installing React router. These essential building blocks will be used to render the product categories, style the product list, and configure the product details page. From there we dive into styled components, refactoring the shop layout, and exploring the concept of "context" in React. In the final stages of the project students will configure the shopping cart basket, and the integrated checkout feature. We will also implement a product search feature, followed by in-depth exercises on validating input forms in React.As you can see this course covers a tremendous bit of ground. Best of all it's authored by Tim Maclachlan - a renowned senior full-stack developer with over 20 years of commercial development experience. As a multi-faceted developer, Tim's core competencies include algorithmic, analytical and mobile development. To date, he's written hundreds of applications and worked in a number of industries from commercial aviation and military, to banking and finance. Tim has a genuine passion for teaching others how to become better coders and looks forward to interacting with his students.With that said, we hope you're just as excited about this course as we are, if so - hit the enroll button and let's get started.
Overview
Section 1: Introduction to React
Lecture 1 Skills Required
Lecture 2 What is React?
Section 2: Project 1: Build a Calculator in React
Lecture 3 Project Overview
Lecture 4 Hello React
Lecture 5 Tools Needed
Lecture 6 Code Pen
Lecture 7 Intro to JSX
Lecture 8 Functional Components in React
Lecture 9 Why Components?
Lecture 10 Intro to Props in React
Lecture 11 React Components, Props and Callbacks
Lecture 12 Building the Calculator Visuals
Lecture 13 onclick Events in React
Lecture 14 Passing Parameters in Callback Functions
Lecture 15 Using React State Hook
Lecture 16 Implementing the calculator - Display
Lecture 17 Implementing the calculator - Operators
Lecture 18 Debugging in React
Lecture 19 Project Summary
Section 3: Project 2 - Build a Connect-4 Clone in React
Lecture 20 Project Introduction - What We Will Build
Lecture 21 Tools Needed
Lecture 22 Creating the Game Board
Lecture 23 Game Circle - onclickEvent
Lecture 24 Passing Props - Destructing - React Children
Lecture 25 Passing Arguments to Click Events
Lecture 26 Inline Styling
Lecture 27 Square to Circle Component
Lecture 28 Global Styling
Lecture 29 Dynamic Styling
Lecture 30 Dynamic Classes
Lecture 31 Handling Callbacks
Lecture 32 Using React State Hook (again)
Lecture 33 Updating the Player Circle
Lecture 34 Initializing the Game Board
Lecture 35 React Key Property
Lecture 36 Styling the Game Board - Header and Footer
Lecture 37 Calculating the Winner
Lecture 38 Displaying the Winner
Lecture 39 Determining a Draw Condition
Lecture 40 React Lifecycle Events
Lecture 41 Initializing the Game
Lecture 42 Suggesting a Move - Implementing a Computer Player
Lecture 43 Smart Computer Player (Basic AI)
Lecture 44 CSS Variables
Lecture 45 Conditional Rendering
Lecture 46 Deploy to Netlify
Lecture 47 Deploy to Surge
Lecture 48 Project Summary
Section 4: Project 3 - Build an E-Commerce Site in React
Lecture 49 Project Introduction - What We Build
Lecture 50 Scaffolding the Project
Lecture 51 Intro to JSON Server
Lecture 52 Fetch API
Lecture 53 Styling the Store
Lecture 54 Rendering the Categories
Lecture 55 Binding the Products
Lecture 56 Refactor the Fetch API call
Lecture 57 Dealing with errors in Fetch API
Lecture 58 Tidy the Fetch API call
Lecture 59 Styling the Product List
Lecture 60 Installing React Router
Lecture 61 Fixing the Key Warning
Lecture 62 React Router - Detail Page
Lecture 63 Fetch API - Get Product By Id
Lecture 64 Product Description
Lecture 65 Intro to Styled Components
Lecture 66 Styled Components - Product Description
Lecture 67 Dangerously Set HTML (Yeah Really)
Lecture 68 Refactor the Categories
Lecture 69 Refactor the Layout
Lecture 70 Refactor the Home Page
Lecture 71 Intro to Context in React
Lecture 72 UseContext Hook and UseReducer Hook in React
Lecture 73 Basket Layout
Lecture 74 Implementing the Basket
Lecture 75 Implementing Basket Icons
Lecture 76 Implementing Basket Total
Lecture 77 Finishing the Checkout
Lecture 78 Checkout - Fixing the State
Lecture 79 Implementing the Order Confirmation
Lecture 80 Intro to Local Storage
Lecture 81 Implementing Search Results
Lecture 82 Better Searching with Debouncing
Lecture 83 Validating Forms in React - Part 1
Lecture 84 Validating Forms in React - Part 2
Lecture 85 Validating Forms in React - Part 3
Lecture 86 Validating Forms in React - Part 4
Lecture 87 Project Summary
Students interested in learning to Build Web Apps using React,Students interested in using jаvascript libraries for Building our Functional UI Components.,Students interested in interactive front-end development using React
Homepage
https://www.udemy.com/course/react-complete-developer-course-with-hands-on-projects/
Download from UploadCloud
https://www.uploadcloud.pro/3lzty0cb80b1/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part5.rar.html
https://www.uploadcloud.pro/hbkdlpw96tii/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part4.rar.html
https://www.uploadcloud.pro/lti9qj7ajnxn/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part3.rar.html
https://www.uploadcloud.pro/mbqgnmtnvwkp/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part1.rar.html
https://www.uploadcloud.pro/zdjgifxesqho/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part2.rar.html

https://rapidgator.net/file/3654d2e8cf2ffb2bbc12dd887e54f3ef/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part1.rar.html
https://rapidgator.net/file/7e3f884fabe96c1251a15c46cf721817/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part5.rar.html
https://rapidgator.net/file/a0bfff81f509e1e6293ec84beef57c66/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part2.rar.html
https://rapidgator.net/file/c566e377de8ca3c29c1aa4be58b32ade/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part4.rar.html
https://rapidgator.net/file/cc22f8c85938e79602717f530828b206/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part3.rar.html

https://uploadgig.com/file/download/6be5e95128b372FB/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part2.rar
https://uploadgig.com/file/download/9D9c9a1420f0f2Cb/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part4.rar
https://uploadgig.com/file/download/A5b597b709fd9273/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part3.rar
https://uploadgig.com/file/download/b9e8f012c6ECb4fd/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part5.rar
https://uploadgig.com/file/download/bB860012ee8b03a5/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part1.rar

https://freshwap.cc/view/3C1F8C245372B4E/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part1.rar
https://freshwap.cc/view/473682A6B011937/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part4.rar
https://freshwap.cc/view/7236C4B5CEC4E93/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part5.rar
https://freshwap.cc/view/B2B7D8866105F15/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part3.rar
https://freshwap.cc/view/D4B2F70629DFCB1/rqzdc.React..Complete.Developer.Course.With.HandsOn.Projects.part2.rar
Views: 13
Comments (0)
free React - Complete Developer Course With Hands-On Projects, Downloads React - Complete Developer Course With Hands-On Projects, RapidShare React - Complete Developer Course With Hands-On Projects, Megaupload React - Complete Developer Course With Hands-On Projects, Mediafire React - Complete Developer Course With Hands-On Projects, DepositFiles React - Complete Developer Course With Hands-On Projects, HotFile React - Complete Developer Course With Hands-On Projects, Uploading React - Complete Developer Course With Hands-On Projects, Easy-Share React - Complete Developer Course With Hands-On Projects, FileFactory React - Complete Developer Course With Hands-On Projects, Vip-File React - Complete Developer Course With Hands-On Projects, Shared React - Complete Developer Course With Hands-On Projects, Please feel free to post your React - Complete Developer Course With Hands-On Projects Download, Movie, Game, Software, Mp3, video, subtitle, sample, torrent, NFO, Crack, uploaded, putlocker, Rapidgator, mediafire, Netload, Zippyshare, Extabit, 4shared, Serial, keygen, Watch online, requirements or whatever-related comments here.
Related Downloads :
{related-news}